How to generate random unique string in PHP
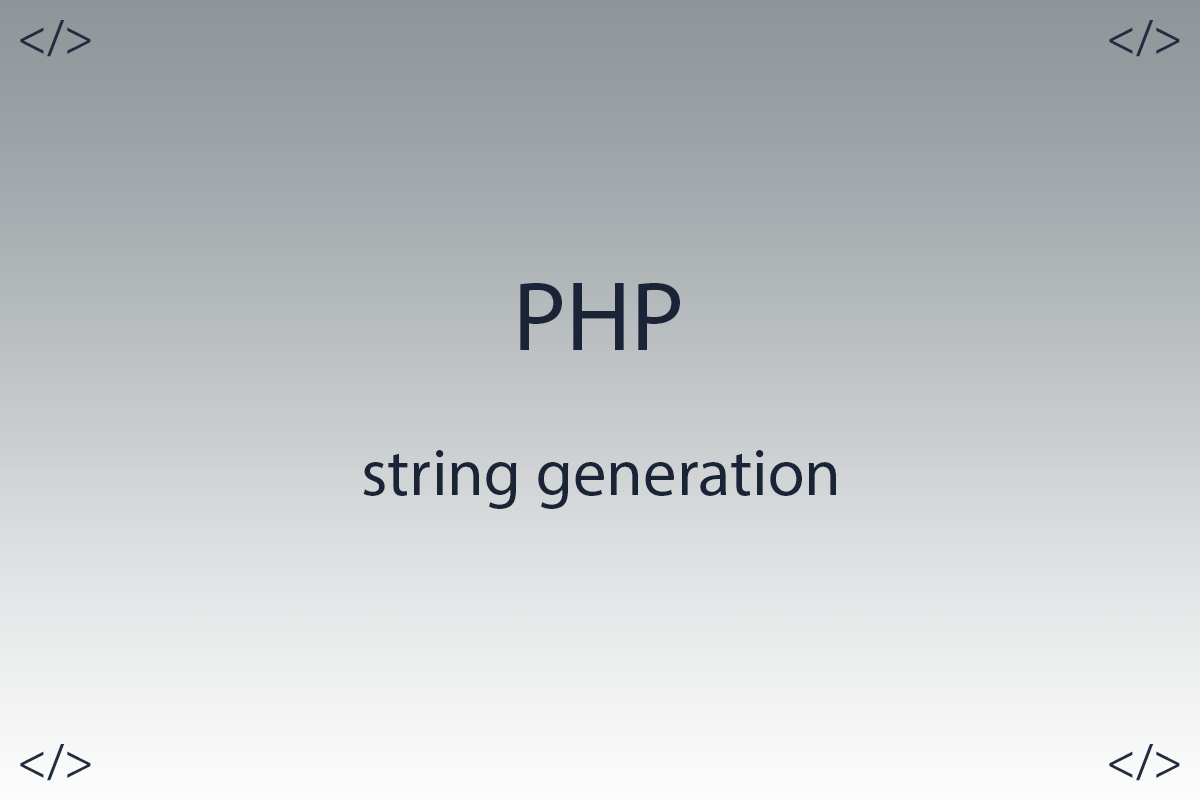
Colleagues hello to all.
In today's article, we'll talk about how to generate a unique string in PHP.
Almost always in your developments you will encounter the fact that you need to generate a unique string of a certain length, and there are a lot of examples of this. One example where you will need to generate a unique string is a cookie for users who register on your site, and this cookie will be used by the user each time they log in.
There are a lot of options in PHP for how to generate a unique string, and I will show you the most common options that developers use.
In the very first option, we will use the uniqid function. The uniqid function generates a unique string based on getting the current time in microseconds. This function has two optional parameters that can be passed, but in order for us to generate a unique string, we can additionally not pass anything.
php> uniqid();
We've called the uniqid function twice, resulting in two unique strings.
In the following example, we will use two functions, random_bytes and bin2hex, to generate a unique string.
php> bin2hex(random_bytes(20));
First, the random_bytes function generates cryptographically pseudo-random bytes, the function takes a mandatory length parameter, that is, the length of the generated string in bytes. After generating a string in bytes by a further action, this string must be passed to the bin2hex function. The bin2hex function is designed to convert binary data to a hexadecimal representation, that is, to a regular string.
The next option to generate a unique string, we will use already three functions, substr, md5 and time.
php> substr(md5(time()), 0, 10);
We first get a set of digits by calling the time function which returns the number of seconds since the Unix epoch. In the next step, we pass this set of numbers to the md5 function, which forms the hash of the string. As a final step, we pass this hash of the string to the substr function, which will truncate the string to the length we want by passing in the second parameter, length.
The next three options are very similar to the previous option for getting a string. All those functions will be used, but only in a different sequence.
php> substr(sha1(time()), 0, 5);
php> md5(time());
php> sha1(time());
As a last option, I will provide you with two of my functions that can generate a string of the length that we want.
First function.
function generateRandomString($length = 20) {
$characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
$charactersLength = strlen($characters);
$randomString = '';
for ($i = 0; $i < $length; $i++) {
$randomString .= $characters[rand(0, $charactersLength - 1)];
}
return $randomString;
}
Second function.
function rand_str() {
$characters = '0123456789ampabcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
$randomstr = '';
for ($i = 0; $i < random_int(10, 30); $i++) {
$randomstr .= $characters[rand(0, strlen($characters) - 1)];
}
return $randomstr;
}
Thank you all, I hope that my article helped you in some way.